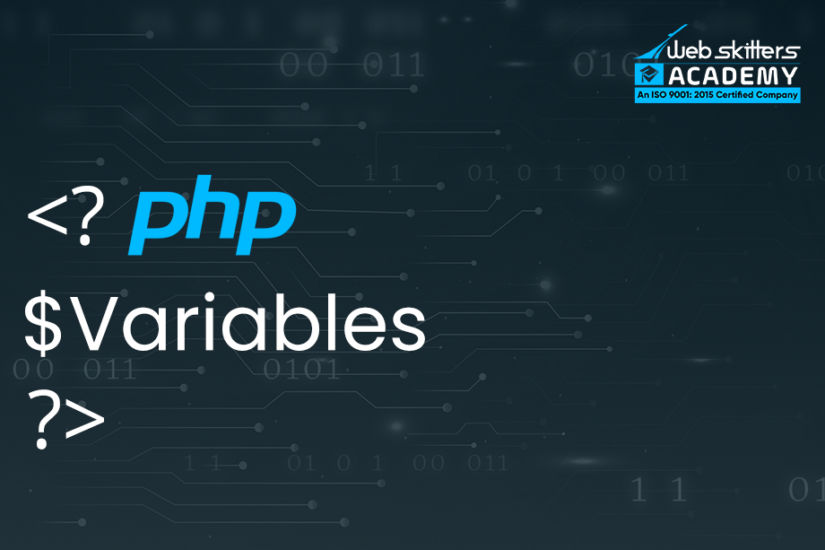
What Is Variable And How To Declare Php Variable?
Table of Contents
Variables are used to store information which can be referenced and manipulated within a computer program. These variables also play a vital role in labeling data with a descriptive name, which makes it easier for the users to understand the program clearly and impressively. Some programmers also consider the variables as containers which can hold information.
In every program, the sole purpose of a variable is to label the data and store it, somewhere in the memory. This data can be used throughout the program, anytime sooner or later.
When it comes to PHP, declaring and storing variables are different. The variables in PHP hold the value or information like string, integar etc. In PHP, a variable is declared by using the “$” sign. It should be followed by the name of the variable.
While declaring variables in PHP, you might have to follow few basic rules, including the following:
- The variable declared in PHP must always start with “$” sign.
- The names of the variable can vary in length- it can be long or short name.
- In PHP, a variable name is only allowed to contain alphanumeric characters and underscores.
- The variables cannot start with a number.
- A variable name must begin either with a letter or an underscore but not integer.
- PHP variable declare only alpha-numeric, character, underscore (A-Z,a-z 0-9, _).
For example: $name=”hello world”;
Here $name is a variable and “hello world” value.
- Case-sensitive variables are the constants used for a simple value that cannot be modified.
- When you get to assign a variable, the assignment is done through an assignment operator, “equal to (=)”.
- The name of the variables are written to the left of the sign and the expression and values are mentioned to the right.
- PHP variables are extremely case-sensitive. For example, it treats $sum and $SUM differently.
- When you declare a variable, no space can be given in the variable name. In case you want to give space, you have to use underscore.
- Let’s see the example to store string, integer, and float values in PHP variables.
Example of how to store string, integer, and float values in PHP variable:
Example 1:
<?php
$string=”hello I am a string variable”; //this is a php string value
$integer=600; // this is a php integer value
$float=50.8; // this is a php float value
echo “this is a string value: $string<br>”;
echo “this is a integer value: $integer<br>”;
echo “this is a float value: $float<br>”;
?>
Output:
this is a string value :hello I am a string variable
this is a integer value: 600
this is a float value: 50.8
Example 2:
Addition of two number-
<?php
$number1=200;
$number2= 300;
$result=$number1+$number2;
Echo “sum of two number is: $result”;
?>
Output:
Sum of two number is: 500
Scope of a variable:
The scope of a variable can be defined as its extent in program. It can be accessed in the program. Easily explained, it is the portion of a program within which it is clearly visible and accessible. PHP has three variable scopes:
Local variable:
These are the variables which are declared within a function. It is so named as it has scope only within that particular function. It is not able to access outside the particular function. Any declaration of a variable that you use outside the function with the similar name, as the one used within the function, becomes a completely different one.
Example:
<?php
$num = 60;
Function local_var()
{
//This $num is local to this function
//the variable $num outside this function
//is a completely different variable
$num = 50;
echo “local num = $num \n”;
}
local_var();
//$num outside function local_var() is a
// completely different variable than that of
// inside local_var()
echo “Variable num outside local_var() is $num \n”;
?>
Output:
Local num = 50
Variable num outside local_var() is 60
Global variable:
The variables that are declared outside a particular function are known as global variables. One can access directly outside that particular function. In order to access the variable within a function, one need to use the word “global”, in front of the variable to refer to the particular global variable.
Example:
<?php
$num = 20;
// functionto demonstrate use of global variable
Function global_var()
{
//we have to use global keyword before
// the variable $num to access within
// the function
global $num;
echo “variable num inside function : $num \n”;
}
global_var();
echo “Variable num outside function : $num \n”;
?>
Output:
Variable num inside function : 20
Variable num outside function : 20
Static variable:
It is the characteristic of PHP to delete a variable. Once the variable completes its execution and the entire memory is freed, it is to be deleted. But sometimes, the variables need to be stored even after the function is executed. To conduct this, PHP developers use static keyword and the variables then are known as the static variables.
Example:
<?php
// function to demonstrate static variables
functionstatic_var()
{
// static variable
static $num = 5;
$sum = 2;
$sum++;
$num++;
echo $num, “\n”;
echo $sum, “\n”;
}
// first function call
static_var();
// second function call
static_var();
?>
Output:
6
3
7
3
Conclusion:
Learn more about PHP variables and broaden your knowledge under the expert guidance at Webskitters Academy! We provide complete training to transform you from a novice to an expert in the market.
Also Read,