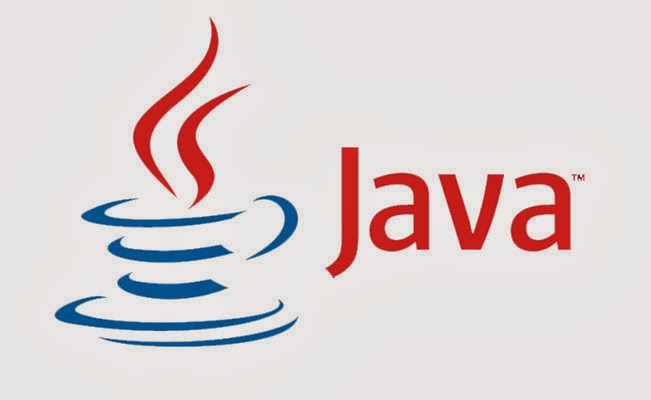
Java Generics programming is introduced in J2SE 5 for dealing with type-safe objects. Before generics, one was able to store any type of objects in the collection which was non-generic. Now with generics, Java programmers are forced to store the specific type of objects. Several abstract data types represent a linear sequence of elements but with general support for removing or adding elements at different arbitrary positions. Designing a simple abstraction is well suited for proper implementation with an array or linked list which can be challenging as there are different nature of data structures.
Various locations within an array can be described by an integer index. An index of an element e in a particular sequence is equal to the number of elements e present in that sequence. The first elements of the sequence have index 0 while the last has index n-1 given the fact that n is the total number of elements. The concept of element’s index is defined for a linked list but it is not such a convenient notion as there is no fixed way to access an element at a given index without traversing a portion of a linked list which depends right on the magnitude of the index.
Java defined an interface, java.util.List, that which will include the following index-based methods:
- Size (): returns the number of elements presents in the list.
- Is Empty(): This will return a Boolean indicating whether the list is empty or not.
- Get(i): This will return the element of the list having the index I; which is an error condition occurring if it is not in the range [0, size()-1].
- Set (i,e): This will replace element at index I with e, and return the old elements which were replaced. Usually, error condition occurs if this is not in the range [0, size ()-1]
- add (i,e): This will insert new element e right into the list so that it has the index I moving all the subsequent elements one index later in the list with the error condition to report.
- Remove (i): This will remove and return the element at index I, moving all elements one index earlier in the list; an error condition will occur if it is not in the range [0, size ()-1].
Indexing of an existing element may change certainly over time as with other elements which are added or removed right in front of it. There are valid ranges for adding method which will include the current size of the list in the case new elements becomes the last elements.
There is the generics framework which is introduced in Java SE 5.0 and is updated in Java SE7 providing support which will allow different parameterization of the types.
The benefit of generics is a very significant reduction in the amount of code which is needed to be written when one is developing a library. There is certainly one more benefit that is eliminating the casting in various situations. There are classes of Collections Framework, the class Class and various other Java libraries which have been updated for including the generics.
Generic Classes and Interfaces:
Right in generic classes and interfaces, parameterization of types can happen by adding a type parameter well within the angular brackets (i.e.,). The type is present at the place of the brackets. Right once it is instantiated, there is generic parameter type which is applied right throughout the class for the various methods which have the same type which is specified. Take the following example, the add() and get() methods which will use parameterized type right as their parameter argument and return types respectively:
public class ListInterface {
public boolean add(E e);
E get(int index);
}
When a variable of a parameterized type is declared, there is concrete type (i.e, < String>) which is specified to be used right in place of the type parameter (i.e. < E>). There is the need to cast the retrieving elements from such things right as collections would surely be eliminated:
public class ListInterface {
List< String > iList = new ArrayList< String >();
iList.add(“Raj”);
// Explicit cast not necessary
String i = iList.get(0);
// Collection List/ArrayList without Generics
List iList = new ArrayList();
iList.add(“Raj”);
// Explicit cast is necessary
String i = (String)iList.get(0);
public class ListInterface {
public interface List {
/∗∗ Returns the number of elements in this list. ∗/
int size();
/∗∗ Returns whether the list is empty. ∗/
boolean isEmpty();
/∗∗ Returns (but does not remove) the element at index i. ∗/
E get(int i) throws IndexOutOfBoundsException;
/∗∗ Replaces the element at index i with e, and returns the replaced element. ∗/
E set(int i, E e) throws IndexOutOfBoundsException;
/∗∗ Inserts element e to be at index i, shifting all subsequent elements later. ∗/
void add(int i, E e) throws IndexOutOfBoundsException;
/∗∗ Removes/returns the element at index i, shifting subsequent elements earlier. ∗/
E remove(int i) throws IndexOutOfBoundsException;
}
Program which implements the performing of the ArrayList
1. Create ArrayList,
2. Insert objects into ArrayList
3. Fetch objects on basis of index,
4. find a search for object in ArrayList,
5. insert a new item at specific index,
6. confirm state of ArrayList ( empty/fill),
7. using Iterator for ArrayList display,
8. find the size of the ArrayList
package listinterface;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Scanner;
public class ListInterface { public static void main(String[] args) { String s; char ch; List myList; // create ArrayList myList=new ArrayList(); Scanner sc=new Scanner(System.in); while(true){ System.out.println("Enter String: "); s=sc.next(); myList.add(s); // insert objects into ArrayList System.out.println("Would you like to Continue: "); ch=sc.next().charAt(0); if(ch=='y') continue; else break; } System.out.println("Fetch objects on basis of index "); for(int c=0;c<myList.size();c++) // find size of the ArrayList System.out.println("Item: "+myList.get(c)); System.out.println("Search an item: "); // find a search for object in ArrayList String search=sc.next(); System.out.println("Index of "+search+" is "+myList.indexOf(search)); System.out.println("Enter new item: "); // insert a new item at specific index String new_intem=sc.next(); System.out.println("Enter position for new item: "); Integer pos=sc.nextInt(); myList.add(pos,new_intem); if(!myList.isEmpty()) // confirm state of ArrayList (empty/fill) { System.out.println("List not empty, so item are: "); for(int c=0;c<myList.size();c++) System.out.println("Item: "+myList.get(c)); } Iterator itr = myList.iterator(); // using Iterator for ArrayList display while(itr.hasNext()){ System.out.println(itr.next()); } } } Also Read, Tutorials Concept On Regular Expressions In Programming Tutorial On The Set Interface On Java
Search
I Want to Learn...
Category
Explore OurAll CoursesTransform Your Dreams
into Reality
Subscribe to Our Newsletter
"*" indicates required fields